Tips
Using Paged.js with Laravel
Using Paged.js with Laravel
Using Paged.js with Laravel
Create a nice PDF with Laravel and Paged.JS
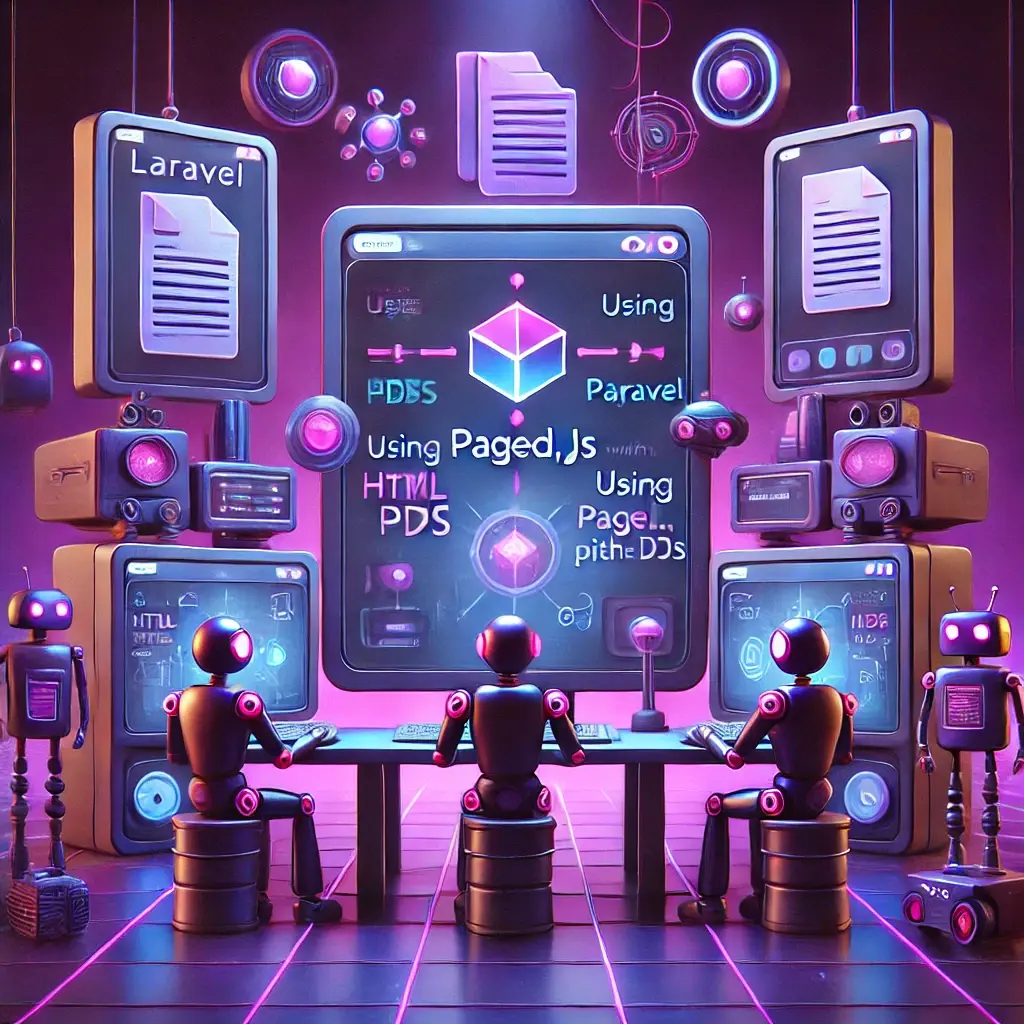
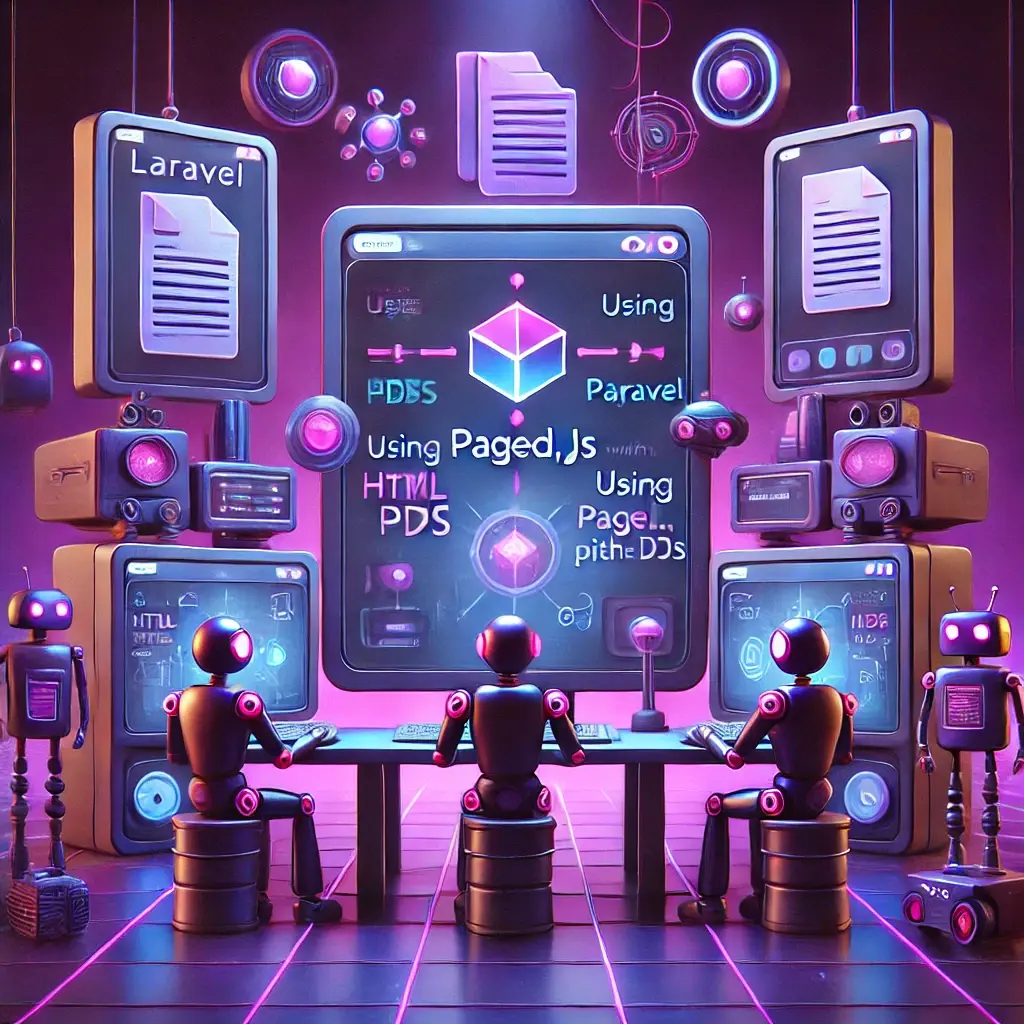
Setting Up a Laravel Project
First, make sure you have PHP and Composer installed. Then create a new Laravel project:
composer create-project laravel/laravel my-amazing-project
cd my-amazing-project
Here is the official documentation.
Create a new View
In Laravel, views are stored in resources/views
and use the Blade templating engine. We can then use controllers to pass data to these views, which generate dynamic HTML for the user's browser.
Let’s create a new file named magazine.blade.php
in the resources/views
directory with the following content:
<!-- resources/views/magazine.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Magazine</title>
</head>
<body>
<div class="content">
<h1 class="main-title">Doppio Magazine</h1>
<section class="page" id="first-page">
<h2 class="title">Chapter 1</h2>
<p class="text">This is the content of the first page.</p>
</section>
<section class="page" id="second-page">
<h2 class="title">Chapter 2</h2>
<p class="text">This is the content of the second page.</p>
</section>
<section class="page" id="third-page">
<h2 class="title">Chapter 3</h2>
<p class="text">This is the content of the third page.</p>
</section>
<section class="page" id="fourth-page">
<h2 class="title">Chapter 4</h2>
<p class="text">This is the content of the fourth page.</p>
</section>
<section class="page" id="fifth-page">
<h2 class="title">Chapter 5</h2>
<p class="text">This is the content of the fifth page.</p>
</section>
</div>
</body>
</html>
Install PagedJS via a CDN
Paged.js can be integrated into a Laravel project in three main ways: using a CDN, installing via NPM (or Yarn), or downloading the library manually.
Including it via a CDN is the simplest method, requiring just a script tag in your Blade template.
This approach offers ease of use, quick updates, reduced server load, and benefits from browser caching.
So we will use this last method ! We just have to include the following script in our view:
<script src="https://unpkg.com/pagedjs/dist/paged.polyfill.js"></script>
Styling the Page
To style a page using Paged.js, we can apply CSS rules to define layout and appearance. Paged.js processes these styles to generate a paginated, print-ready layout.
We can also use specific Paged.js CSS features like @page
and @media print
for pagination and print styling.
Let’s design our magazine style ! Into the /public/css
folder, we have to create a magazine.css
file:
/* public/css/magazine.css */
.content {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100%;
padding: 2rem 4rem;
box-shadow: 0 0 0 1px grey;
}
.page {
break-after: page;
}
h1 {
text-align: center;
color: #5935dd;
}
h2 {
text-align: center;
color: #3c3c3c;
}
p {
text-align: center;
color: #a1a1a1;
}
Then, we have to import the .css file in our view by adding the stylesheet into the <head> tag:
<head>
<link rel="stylesheet" href="{{ asset('css/magazine.css') }}">
</head>
Finally, our view should look like this:
<!-- resources/views/magazine.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Magazine</title>
<link rel="stylesheet" href="{{ asset('css/magazine.css') }}">
</head>
<body>
<div class="content">
<h1 class="main-title">Doppio Magazine</h1>
<section class="page" id="first-page">
<h2 class="title">Chapter 1</h2>
<p class="text">This is the content of the first page.</p>
</section>
<section class="page" id="second-page">
<h2 class="title">Chapter 2</h2>
<p class="text">This is the content of the second page.</p>
</section>
<section class="page" id="third-page">
<h2 class="title">Chapter 3</h2>
<p class="text">This is the content of the third page.</p>
</section>
<section class="page" id="fourth-page">
<h2 class="title">Chapter 4</h2>
<p class="text">This is the content of the fourth page.</p>
</section>
<section class="page" id="fifth-page">
<h2 class="title">Chapter 5</h2>
<p class="text">This is the content of the fifth page.</p>
</section>
</div>
<script src="https://unpkg.com/pagedjs/dist/paged.polyfill.js"></script>
</body>
</html>
Configure the Route
Next, we need to set up a route to handle the display of our magazine view. Open routes/web.php
and add the following route:
// routes/web.php
use Illuminate\Support\Facades\Route;
Route::get('/magazine', function () {
return view('magazine');
});
Display custom content through "named strings"
What if we want to display the name of the chapter we are in at the bottom of the PDF ?
To do that, let's use the specific Paged.js CSS features @page
into our style file:
/* public/css/magazine.css */
@page {
@bottom-center { content: string(title); }
}
.chapter > h2 {
string-set: title content(text);
}
The first block, is used to tell PagedJS to add content in the margin of the PDF, at the bottom center position (check all the positions available).
The second block, is used to make available the title of the chapter to PagedJS, we use the named strings feature from PagedJS to give the content of the h2 tag from every chapter into the named string "title".
Generate PDFs
Now that you have created a webpage that displays as a PDF using Paged.js, you can easily generate PDF files out of this page using Doppio.
What's next ?
Be sure to check out the Paged.js documentation to see all the features available and how you can use them to render beautiful PDFs !
Setting Up a Laravel Project
First, make sure you have PHP and Composer installed. Then create a new Laravel project:
composer create-project laravel/laravel my-amazing-project
cd my-amazing-project
Here is the official documentation.
Create a new View
In Laravel, views are stored in resources/views
and use the Blade templating engine. We can then use controllers to pass data to these views, which generate dynamic HTML for the user's browser.
Let’s create a new file named magazine.blade.php
in the resources/views
directory with the following content:
<!-- resources/views/magazine.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Magazine</title>
</head>
<body>
<div class="content">
<h1 class="main-title">Doppio Magazine</h1>
<section class="page" id="first-page">
<h2 class="title">Chapter 1</h2>
<p class="text">This is the content of the first page.</p>
</section>
<section class="page" id="second-page">
<h2 class="title">Chapter 2</h2>
<p class="text">This is the content of the second page.</p>
</section>
<section class="page" id="third-page">
<h2 class="title">Chapter 3</h2>
<p class="text">This is the content of the third page.</p>
</section>
<section class="page" id="fourth-page">
<h2 class="title">Chapter 4</h2>
<p class="text">This is the content of the fourth page.</p>
</section>
<section class="page" id="fifth-page">
<h2 class="title">Chapter 5</h2>
<p class="text">This is the content of the fifth page.</p>
</section>
</div>
</body>
</html>
Install PagedJS via a CDN
Paged.js can be integrated into a Laravel project in three main ways: using a CDN, installing via NPM (or Yarn), or downloading the library manually.
Including it via a CDN is the simplest method, requiring just a script tag in your Blade template.
This approach offers ease of use, quick updates, reduced server load, and benefits from browser caching.
So we will use this last method ! We just have to include the following script in our view:
<script src="https://unpkg.com/pagedjs/dist/paged.polyfill.js"></script>
Styling the Page
To style a page using Paged.js, we can apply CSS rules to define layout and appearance. Paged.js processes these styles to generate a paginated, print-ready layout.
We can also use specific Paged.js CSS features like @page
and @media print
for pagination and print styling.
Let’s design our magazine style ! Into the /public/css
folder, we have to create a magazine.css
file:
/* public/css/magazine.css */
.content {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100%;
padding: 2rem 4rem;
box-shadow: 0 0 0 1px grey;
}
.page {
break-after: page;
}
h1 {
text-align: center;
color: #5935dd;
}
h2 {
text-align: center;
color: #3c3c3c;
}
p {
text-align: center;
color: #a1a1a1;
}
Then, we have to import the .css file in our view by adding the stylesheet into the <head> tag:
<head>
<link rel="stylesheet" href="{{ asset('css/magazine.css') }}">
</head>
Finally, our view should look like this:
<!-- resources/views/magazine.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Magazine</title>
<link rel="stylesheet" href="{{ asset('css/magazine.css') }}">
</head>
<body>
<div class="content">
<h1 class="main-title">Doppio Magazine</h1>
<section class="page" id="first-page">
<h2 class="title">Chapter 1</h2>
<p class="text">This is the content of the first page.</p>
</section>
<section class="page" id="second-page">
<h2 class="title">Chapter 2</h2>
<p class="text">This is the content of the second page.</p>
</section>
<section class="page" id="third-page">
<h2 class="title">Chapter 3</h2>
<p class="text">This is the content of the third page.</p>
</section>
<section class="page" id="fourth-page">
<h2 class="title">Chapter 4</h2>
<p class="text">This is the content of the fourth page.</p>
</section>
<section class="page" id="fifth-page">
<h2 class="title">Chapter 5</h2>
<p class="text">This is the content of the fifth page.</p>
</section>
</div>
<script src="https://unpkg.com/pagedjs/dist/paged.polyfill.js"></script>
</body>
</html>
Configure the Route
Next, we need to set up a route to handle the display of our magazine view. Open routes/web.php
and add the following route:
// routes/web.php
use Illuminate\Support\Facades\Route;
Route::get('/magazine', function () {
return view('magazine');
});
Display custom content through "named strings"
What if we want to display the name of the chapter we are in at the bottom of the PDF ?
To do that, let's use the specific Paged.js CSS features @page
into our style file:
/* public/css/magazine.css */
@page {
@bottom-center { content: string(title); }
}
.chapter > h2 {
string-set: title content(text);
}
The first block, is used to tell PagedJS to add content in the margin of the PDF, at the bottom center position (check all the positions available).
The second block, is used to make available the title of the chapter to PagedJS, we use the named strings feature from PagedJS to give the content of the h2 tag from every chapter into the named string "title".
Generate PDFs
Now that you have created a webpage that displays as a PDF using Paged.js, you can easily generate PDF files out of this page using Doppio.
What's next ?
Be sure to check out the Paged.js documentation to see all the features available and how you can use them to render beautiful PDFs !
By Doppio
June 11, 2024